Guide to Analyzing Programs Written in Visual Basic 6.0
What Will Be Covered
Within the programming community, there is a belief that Visual Basic is a terrible programming language. This has spawned numerous wildly varying myths and legends surrounding it. Some say it cannot compile programs into machine code. Others claim it cannot work with memory addresses. Still others write that it does not allow inserting assembly procedures into the high-level code of the program. However, all these myths are blatant lies and the incompetence of those who have looked at this programming language from afar, could not find similar to C++ methods for implementing required tasks, and loudly decided that Visual Basic is incapable of more than teaching students programming. In reality, starting with version 5.0, this language allows compiling programs into full-fledged machine Native Code. There is also the possibility of working with memory addresses, for which there are functions VarPtr and StrPtr. Assembly procedures can also be used. Not as easily as in Delphi and C++, but still. As we can see, Visual Basic does not have too many drawbacks, and its advantages are so numerous that they cannot be described even in several articles.
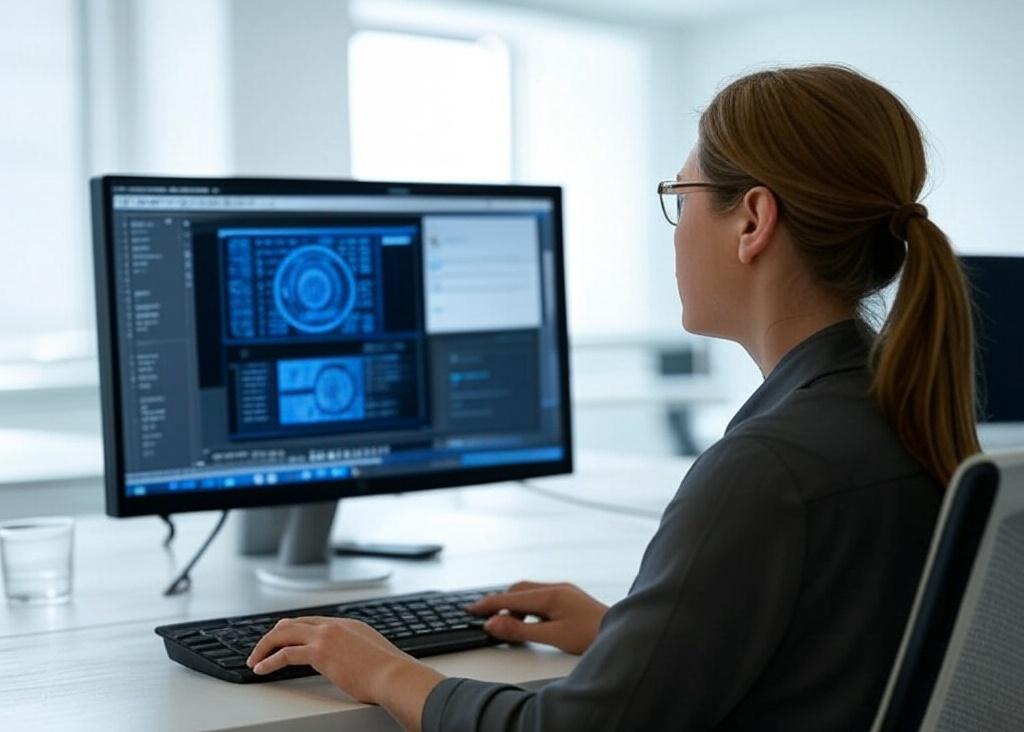
For now, I will only talk about the most obvious and important ones:
- Full-fledged compilation and small size of resulting EXE files. Despite requiring the MSVBVM60.DLL library, this is not a drawback as it is integrated into all new operating systems and there is no need to supply it together with the application. In fact, even in such cases its size is only 1.3Mb, which is negligible.
- Ease of writing code. Everything is convenient and visual. Even a beginner can write non-optimized but functional programs. As they say: if you know how to write quality - write, as the programs will only get better. If not - you can write non-optimized programs without declaring variables (it's not necessary), without converting data types (it's lso not necessary) and so on. And everything will work perfectly, albeit not significantly slower than after compiling programs written according to all the rules of proper development. Even as a novice, deeper knowledge will gradually come to you, and you will start developing large projects that are no worse than analogs in popular C/C++-like languages.
Reverse medal of unpopularity for Visual Basic is that researchers mistakenly believe that programs written in Visual Basic are absolutely impossible to analyze. If the program has been compiled into P-Code (VB6 pseudocode of virtual machine) - until 2005 it it could be partially true, when there were no available decompilers like for example VB Decompiler. But even back then, an debugger (albeit often prone to errors) and P-Code disassemblers (of roughly similar quality) had already been written, and working with code was already feasible, albeit difficult. Plus, I also wrote the article Decompiling P-Code in Your Mind's Eye - The Subtleties of Researching Commands of the VB Virtual Machine, which answers many questions about working with P-Code.
As for programs compiled into Visual Basic in machine Native Code, they are analyzed almost the same way as any x86 software code, written for example in C++ or Delphi. There are, however, a number of peculiarities that should be mentioned separately. All operations performed by the program use specialized functions from the MSVBVM60.DLL library. The names of many of these functions resemble Visual Basic operators, so looking at the names of some of them can give you an idea of what operations they perform. There are, of course, also variants that do not always follow logic. I will tell you about them in this article.
Functions of the Visual Basic 6.0 library
The most confusing functions for analysts are usually those related to data conversion from one type to another. Below, I provide a list of existing types of data used in Visual Basic 6.0 and their corresponding functions for working with them for you convenience.
bool - boolean
str - string
i2 - integer (a 2-byte integer)
ui2 - unsigned integer (a 2-byte unsigned integer)
i4 - long (a 4-byte integer)
r4 - single (a 4-byte floating-point number)
r8 - double (an 8-byte floating-point number)
cy - currency (analogous to double but with a currency tie-in)
var - variant (VB) or variable (OLEAUT)
Names of parts that make up function names:
fp - working with float data, passed through ST registers or saved using coprocessor commands
cmp - comparing arguments
comp - also comparing arguments
Functions for data type conversion:
__vbaI2Str - converts String to Integer
__vbaI4Str - converts String to Long
__vbar4Str - converts String to Single
__vbar8Str - converts String to Double
VarCyFromStr - converts String to Currency
VarBstrFromI2 - converts Integer to String
Data transfer
__vbaStrCopy - copies a string to memory, analogous to the API function HMEMCPY
__vbaVarCopy - copies a variable type (Variant) to memory
__vbaVarMove - moves a variable type (Variant) to memory
Mathematical Functions
__vbavaradd - adds two Variant type variables
__vbavarsub - subtracts two Variant type variables
__vbarmul - multiplies two Variant type variables
__vbavardiv - divides two Variant type variables
'with the result outputted to an Integer variable
__vbavarxor - logical XOR operation
Other Functions
__vbavarfornext - used for initializing a For ... Next loop
__vbafreestr - free memory for a string variable
__vbafreeobj - free memory for an object
__vbastrvarval - retrieves numerical value from a string
multibytetowidechar - character encoding conversion
rtcMsgBox - displays a message, similar to the API function MessageBox/A/Ex
__vbavarcat - concatenates two Variant type variables
__vbafreevar - free memory for Variant type variable
__vbaobjset - creates an object
__vbaLenBstr - determines the length of a string
rtcInputBox - displays a form with input field (also may uses API functions GetWindowText/A, GetDlgItemText/A)
__vbaNew - creates a new object
__vbaNew2 - another variant of creating a new object
rtcTrimBstr - removes spaces at the beginning and end of a string
Comparison Functions
__vbastrcomp - compares two string Variables, similar to the API function lstrcmp
__vbastrcmp - compares two string Variables, similar to the API function lstrcmp
__vbavartsteq - compares two Variant type variables
__vbaFpCmpCy - compares a floating-point value with a Currency value
Unlocking Form Control Elements
Any control element on a form can be visible or invisible, accessible or locked. The function __vbaObjSet is used to set object properties. This function is specifically used to lock or unlock control elements on a form or change any of its properties. When analyzing the code, we need to catch the call for this particular function. To do this, open any debugger, such as Olly Debugger, find the __vbaObjSet function in the program's import table, and then set a breakpoint by pressing F2. After that, run the file being investigated.
When the breakpoint triggers, examine the surrounding code. If it resembles
50 push eax 52 push edx FFD7 call edi 8BD8 mov ebx, eax 6A00 push 00 53 push ebx 8B03 mov eax, dword ptr [ebx]
then you are on the right way and can continue your investigation. What do you think this "push 00" is? 00h when passing boolean values in Visual Basic represents False, while FFh (255) represents True. This means that with such code, the current command sets a property of some control element on the form to False. This can mean locking or disabling visibility of a specific control element on the form.
Of course, this could also be setting any other property of the form to True. Since the syntax is the same, you can only determine whether this command relates to changing the lock property by analyzing the surrounding code. However, I believe you will manage it yourself or use VB Decompiler, which unlike OllyDbg is capable of decompiling code and displays machine assembly language instead of a vb-like syntax with type and property decompilation of objects on the form.
Methodology for Analyzing Simple String Data Validation Checks
The following text is partially based on a rather old but relevant article titled How to Research Visual Basic. It was available on the website infonegocio.com long ago, but it has been gone for quite some time now.
What might we need? Any disassembler/debugger. A modern debugger - Olly Debugger, IDA Pro, or even an ancient Win32Dasm will do. If you are using the latter, you can view the functions used by the program in the "Functions" menu -> "Imports".
If you plan to investigate code working with databases, remember that function names don't speak for themselves and it will be difficult to determine what action a particular one performs. In this case, all you can do is look at the names of functions that may have been left by the author of the code. There are not many vulnerabilities in database drivers, so investigating such code can be quite challenging. Again, if you don't use VB Decompiler, but manually disassemble this code in a debugger.
Let's consider an example of code for checking string data and performing/not performing certain functionality based on the results of such comparison. For easy searching, set MessageBox. Open Visual Basic IDE (it will be useful to us later for compiling examples), add a text box to the form and a button, then write the following code in the button's click handler:
Private Sub Command1_Click() Dim X As Integer X = 43690 MsgBox "Checking value" If CLng(Trim$(Text1.Text)) = X Then MsgBox ("Complete") End Sub
Now let's open Olly Debugger, load the file (previously compiled in Visual Basic 6.0) and search for a call to the rtcMsgBox function.
00401F54 FF1520104000 CALL [MSVBVM60!rtcMsgBox] ; MsgBox "Checking value" 00401F5A 8D459C LEA EAX,[EBP-64] 00401F5D 8D4DAC LEA ECX,[EBP-54] 00401F60 50 PUSH EAX 00401F61 8D55BC LEA EDX,[EBP-44] 00401F64 51 PUSH ECX 00401F65 8D45CCLEA EAX,[EBP-34] 00401F68 52 PUSH EDX 00401F69 50 PUSH EAX 00401F6A 6A04 PUSH 04 00401F6C FF1508104000 CALL [MSVBVM60!__vbaFreeVarList] 00401F72 8B0E MOV ECX,[ESI] 00401F74 83C414 ADD ESP,14 00401F77 56 PUSH ESI 00401F78 FF9104030000 CALL [ECX+00000304] 00401F7E 8D55DCLEA EDX,[EBP-24] 00401F81 50 PUSH EAX 00401F82 52 PUSH EDX 00401F83 FF1524104000 CALL [MSVBVM60!__vbaObjSet] 00401F89 8BF0 MOV ESI,EAX 00401F8B 8D4DE4LEA ECX,[EBP-1C] 00401F8E 51 PUSH ECX 00401F8F 56 PUSH ESI 00401F90 8B06 MOV EAX,[ESI] 00401F92 FF90A0000000 CALL [EAX+000000A0] 00401F98 3BC7 CMP EAX,EDI 00401F9A DBE2 FCLEX 00401F9C 7D12 JGE 00401FB0 00401F9E 68A0000000 PUSH 000000A0 00401FA3 6804184000 PUSH 00401804 00401FA8 56 PUSH ESI 00401FA9 50 PUSH EAX 00401FAA FF1518104000 CALL [MSVBVM60!__vbaHresultCheckObj] ; Get contents of Text1.Text 00401FB0 8B55E4MOV EDX,[EBP-1C] 00401FB3 52 PUSH EDX 00401FB4 FF1514104000 CALL [MSVBVM60!rtcTrimBstr] ; Trim$ 00401FBA 8BD0 MOV EDX,EAX 00401FBC 8D4DE0 LEA ECX,[EBP-20] 00401FBF FF1584104000 CALL [MSVBVM60!__vbaStrMove] 00401FC5 50 PUSH EAX 00401FC6 FF1568104000 CALL [MSVBVM60!__vbaI4Str] ; CLng 00401FCC 33C9 XOR ECX,ECX 00401FCE 3DAAAA0000 CMP EAX,0000AAAA ; Integer 43690 in HEX 00401FD3 8D55E0LEA EDX,[EBP-20] 00401FD6 8D45E4LEA EAX,[EBP-1C] 00401FD9 0F94C1 SETZ CL 00401FDC 52 PUSH EDX 00401FDD 50 PUSH EAX 00401FDE F7D9 NEG ECX 00401FE0 6A02 PUSH 02 00401FE2 8BF1 MOV ESI,ECX 00401FE4 FF156C104000 CALL [MSVBVM60!__vbaFreeStrList] 00401FEA 83C40C ADD ESP,0C 00401FED 8D4DDC LEA ECX,[EBP-24] 00401FF0 FF1594104000 CALL [MSVBVM60!__vbaFreeObj] 00401FF6 663BF7 CMP SI,DI 00401FF9 7463 JZ 0040205E ; Jump to the Complete code (MsgBox "Complete") 00401FFB B804000280 MOV EAX,80020004 00402000 8D558C LEA EDX,[EBP-74] 00402003 8D4DCCLEA ECX,[EBP-34] 00402006 8945A4 MOV [EBP-5C],EAX 00402009 895D9C MOV [EBP-64],EBX 0040200C 8945B4 MOV [EBP-4C],EAX 0040200F 895DAC MOV [EBP-54],EBX 00402012 8945C4 MOV [EBP-3C],EAX 00402015 895DBC MOV [EBP-44],EBX 00402018 C7459418184000 MOV DWORD PTR [EBP-6C],00401818 0040201F C7458C08000000 MOV DWORD PTR [EBP-74],00000008 00402026 FF157C104000 CALL [MSVBVM60!__vbaVarDup] 0040202C 8D4D9C LEA ECX,[EBP-64]
To remove the value check, simply change the conditional jump at address 00401FF9 to an unconditional jump. This involves replacing JE with JMP (i.e., 74h with EBh). As we can see, such simple checks in Visual Basic are not very efficient, just like their counterparts in C++. Therefore, if the topic is about checking a serial number or tying software to a disk, developers should strongly consider protecting this code. I recommend using DotFix NiceProtect as one of the good options for such protection. It can both virtualize critical code and protect the program from analysis in general.
Analyzing values in various data types
Below are several methods for efficiently analyzing all sorts of values in Visual Basic code.
Obtaining data if they lie as a string in plain view
When Basic copies a string to memory, you can always intercept it during debugging.
To do this, set a breakpoint on the function __vbaStrCopy and when it triggers for you, just check the code after je 66047B00 (which is contained in the library and identical for all VB6 programs). Then look at the content of register edx minus 4 bytes. In this case, you will see a string that the function is currently working with.
Exported fn(): __vbaStrCopy - Ord:008Ah :66024532 56 PUSH ESI :66024533 57 PUSH EDI :66024534 85D2 TEST EDX, EDX :66024536 8BF9 MOV EDI, ECX :66024538 0F84C2350200 JE 66047B00 :6602453E FF72FC PUSH [EDX-04] ; There is a string at this address :66024541 52 PUSH EDX
Obtaining a string if it is compared to the input
Set a breakpoint at the function __vbaStrComp, and just above its call, there will be two push operations. These push Variant variables for comparison into the stack. Check the contents of register eax to get the address of the text. Usually, this is where you'll find the string we are looking for.
00401BC7 50 PUSH EAX ; The string you entered 00401BC8 6880174000 PUSH 00401780 ; The string we are looking for 00401BCD FF1530104000 CALL [MSVBVM60!__vbaStrComp]
Obtaining data if there is a comparison of 4-byte numerical variables
Set a breakpoint at the function __vbaI4Str. This function is used by the program to convert the input text string into a 4-byte number. When the breakpoint triggers, look at the code below; there will be a comparison procedure for strings. However, the value we need will be in hexadecimal format (e.g., decimal number 987654321 is equal to the hexadecimal number 3ADE68B1).
00401B77 FF155C104000 CALL [MSVBVM60!__vbaI4Str] 00401B7D 8D4DE0 LEA ECX, [EBP-20] 00401B80 8BF8 MOV EDI, EAX 00401B82 FF157C104000 CALL [MSVBVM60!__vbaFreeStr] 00401B88 8D4DDC LEA ECX, [EBP-24] 00401B8B FF1580104000 CALL [MSVBVM60!__vbaFreeObj] 00401B91 81FFB168DE3A CMP EDI, 3ADE68B1 ; 3ADE68B1 - the value we are looking for. 00401B97 7520 JNZ 00401BB9 ; The function will return zero if the input ; and comparison values are equal. ; Therefore, this jump will only work if the ; compared data is different.
Here we can see the correct value or disable the check altogether (to do this, replace JNZ with NOP). As we can see, in this case, you need to change the bytes at address 00401B97 from 7520 to 9090.
Obtaining data during comparison of two-byte numbers
The difference from the previous example is that you need to set a breakpoint on the function __vbaI2Str. Sometimes, however, the string is first converted to a 4-byte number, and then the 4-byte number is converted to a two-byte one using the function __vbaI2I4.
Comparison of two Single type variables
This check can also be easily bypassed. Set a breakpoint on the function __vbaR4Str, and when it triggers, scroll through the machine code below. You will see a jump after the comparison. To invert the condition of the jump, simply change the condition to its opposite.
00401C0C FF153C104000 CALL [MSVBVM60!_vbaR4Str] 00401C12 D95DE4 FSTP REAL4 PTR [EBP-1C] 00401C15 8D4DDC LEA ECX, [EBP-24] 00401C18 8D55E0 LEA EDX, [EBP-20] 00401C1B 51 PUSH ECX 00401C1C 52 PUSH EDX 00401C1D 6A02 PUSH 02 00401C1F FF1570104000 CALL [MSVBVM60!__vbaFreeStrList] 00401C25 83C40C ADD ESP, 0C 00401C28 8D4DD8 LEA ECX, [EBP-28] 00401C2B FF1594104000 CALL [MSVBVM60!__vbaFreeObj] 00401C31 817DE43A92FC42 CMP DWORD PTR [EBP-1C], 42FC923A 00401C38 7520 JNZ 00401C5A ; If zero then entered incorrect value
Replace the instruction at address 00401C38 with 9090 (so that no operations are executed in this place) or change the conditional jump to its opposite. On Visual Basic, you cannot check what you replaced these bytes with as NOP's (9090). If you still have concerns that the code will stop working without commands, simply enter commands that do not make any changes, for example:
inc eax dec eax
These instructions are one-byte and will fit in place of the conditional jump.
ByPassing Float Value Checks (double)
This can also be done very easily. Set a breakpoint at the function __vbaR8Str and scroll through the code slightly below the breakpoint, you will see a comparison procedure (FCOMP REAL8 PTR [Address]), followed by test and jmp. The condition of this jump can also be easily changed to its opposite.
00401C55 CALL [MSVBVM60!rtcTrimBstr] 00401C5B MOV EDX, EAX 00401C5D LEA ECX, DWORD PTR [EBP-28] 00401C60 CALL [MSVBVM60!__vbaStrMove] 00401C66 PUSH EAX 00401C67 CALL [MSVBVM60!__vbaR8Str] 00401C6D FCOMP REAL8 PTR [004010D8]; Comparison of values 00401C73 FSTSW AX ; Processor handling 00401C75 TEST AH, 40 ; Check for correctness of values 00401C78 JE 00401C83 ; Jump
Replace the instruction at address 00401C78 with 9090 (so that no operations are performed in this area) or change the conditional jump to its opposite. As mentioned earlier, you cannot check what you replaced these bytes with as NOP's on Visual Basic. Only external protection can avoid this modifications.
Obtaining a string after XOR-ing it
The algorithm for working with the Visual Basic procedure xor often looks like this. The input string is converted into ASCII codes character by character, which are then XOR'd one at a time. After that, there is an inverse conversion, possibly followed by some mathematical manipulations of the ASCII codes. ANSI codes may be XOR'd with a random or fixed number.
00401E6C 50 PUSH EAX 00401E6D FF1544104000 CALL [MSVBVM60!rtcMidCharBstr] 00401E73 8BD0 MOV EDX, EAX 00401E75 8D4DC8 LEA ECX, [EBP-38] 00401E78 FFD6 CALL ESI 00401E7A 50 PUSH EAX 00401E7B FF1518104000 CALL [MSVBVM60!rtcAnsiValueBstr] 00401E81 0FBFC8 MOVSX ECX, AX 00401E84 81F191000000 XOR ECX, 00000091 ; Ansi char xor'd with 91 00401E8A 51 PUSH ECX ; Result of the xor operation 00401E8B FF1570104000 CALL [MSVBVM60!rtcBstrFromAnsi]
Place a breakpoint on 00401E8A 51 PUSH ECX and monitor the changes in the ECX register content as the cycle is processed. When the program has completed the entire loop - you will have obtained the decrypted string.
CALL [MSVBVM60!rtcMidCharBstr] ; Gets one character from the string CALL [MSVBVM60!rtcAnsiValueBstr] ; Gets an Ansi code from the character CALL [MSVBVM60!rtcBstrFromAnsi] ; Converts the Ansi code to a string
In this case, following the code below will most likely result in a comparison and jump. In this case, CALL [MSVBVM60!__vbaStrComp] may be used for string comparisons. If there is no reference to MSVBVM60!rtcBstrFromAnsi, then certain mathematical manipulations are performed on the string, which can be represented as a 2-byte or 4-byte integer or floating point value. These cases have already been mentioned above.
Conclusion
I hope that after reading this article, Visual Basic programs no longer scare you and their analysis is approximately understood. To see how a Visual Basic code looks in real-time, I recommend checking out other articles on this website here.
Thank you for your interest in this article!
March 25, 2025 (deep rewrite of my early article from September 15, 2004)
(C) Sergey Chubchenko, VB Decompiler's main developer